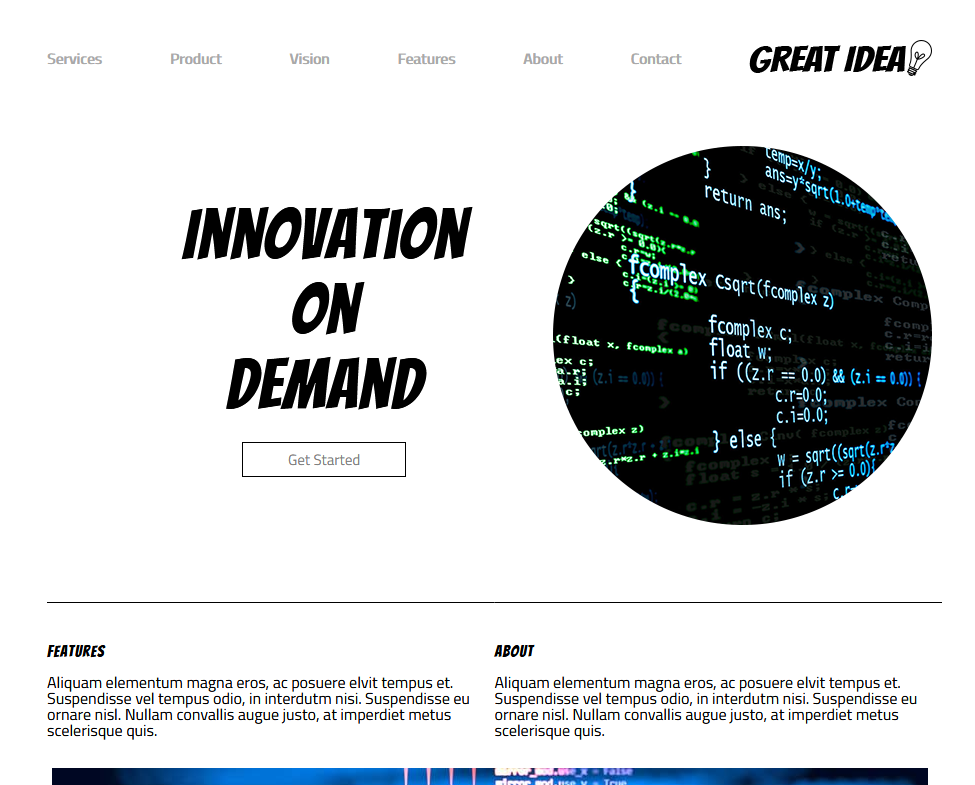
Responsive Design Web Page
In this project, the html was provided and I designed the page by writing CSS. My CSS demonstrates the following:
In this project, the html was provided and I designed the page by writing CSS. My CSS demonstrates the following:
I worked with a partner to design a landing page for the fictitious AtoZ Experiences company. My contribution was the navigation bar, footer, Safety page, and the Contact page. This project demonstrates:
Here I used JavaScript to manipulate the DOM, create components, and wrote event listeners for the page. This project demonstrates:
Components were created with JavaScript and data from a 3rd party library was inserted into these components. This project demonstrates:
In this project, I created a React App calculator by importing data and passing it down to components. This project demonstrates:
In this react App, I created states of data by using the useState hook. The team name, team scores, and seconds are the state values. Buttons were added that allow you to change the state of each of these values. This project demonstrates:
This React App makes an API call to retrieve Star Wars data. This data is then passed down to smaller components that render different parts of the page. Character data is displayed in cards on the page. Some of the data displayed (like home planet) requires additional API calls to retrieve. Separate components are designed to collect this additional data. Buttons were also created to change which page of the character list that you are looking at. This project demonstrates:
I created a react application that mimics Apple's navigation bar and sub-navigation bars. This project demonstrates:
The team builder App had me creating forms to collect and save new data to state. I used HTML form, input, and submit to create the forms. I then wrote functions that would save values to state when the event was triggered. This project demonstrates:
This user on-boarding App uses a React form library called Formik. I created a form with several input fields. I also added error messages incase a form was submitted with missing values. When the submit button is clicked, an API post request sends out the data and receives a response. After a response is received, the name of the user is added to the page to indicate that their information is now saved. This project demonstrates:
This react application uses an API to design a fan page for the Ricky and Morty show. This project demonstrates:
This was my second group project and my first time using React to design components of a much bigger app.
My focus was on the doctor homepage. My task was to pull in patient data and displayed a list of patients on the doctor's dashboard.
The page has a search bar where the doctor can search for patients by name to find them more easily.
Also, the doctor has the ability to click on a patient's 'Records' button to get more information on that patient.
After clicking on the 'Records' button, the doctor is taken to the Single Patient page.
Here all of the patient's immunization information is listed for the doctor.
Also, a button was added that take the doctor to a form that allows them to add a medical record to the database.
A separate component was created which is an indicator button that changes color depending on whether a patient is due a shot or not.
On our Trello Board, you can see that I also
did work on the introduction page and the footer.
To login in as a doctor, the email address is provider1@test.com and the password is 1234.
This project demonstrates:
A React application that uses React classes to take data from an input field and set it to state. Also added the ability to remove selected items from state. This project demonstrates:
This is a React application that demonstrates React component lifecycles. During the mounting phase, an API call is made to collect user information. Upon clicking any of the buttons, the updating phase is initiated and the page is rerendered. Additional features include a search bar that will search Github user names and the Github Contribution Graph. This project demonstrates:
This React app first collects data via an API call to a simple backend that I created. Each player's information is then displayed as a list. I also created two custom hooks. The first allows the user to change the display to dark mode and the second saves the users dark mode choice into local storage. This project demonstrates:
This is not a school project. During a break in classes, I decided to build a react-app to stay in practice. I found a free sports api and built an app based on the NFL. This project demonstrates:
This App displays a component with a car for sale's basic information on the left. On the right was a features component where a user can add features to the car. My task was to implement a global state by using Redux. I also created actions and reducers to change the state, which rerenders the page. The user is able to add and remove features with the price updating with each change. This project demonstrates:
'Dogs' is a react-redux app that makes axios calls from a dog API and displays the response. This project demonstrates:
This is a single page App with different routes that uses Context API to keep a global state. Two different contexts are created. The first context is for the product information and the second is for the shopping cart. The product page and the shopping cart page are given different components that are given different routes. This project demonstrates:
Here I am using Redux as my state management system. I created different actions to collect, submit, and remove data from the backend. I used Axios to make my get, post, and delete calls to the backend. I used useState to save data that was submitted on the pages form inputs before posting it to the backend. This project demonstrates:
I created a database with a server and deployed it on Heroku. The Friends app makes api calls to this database. To keep information private, a log-in with password was created. (User name is 'Lambda School' and password is 'i<3Lambd4'.) This project demonstrates:
This app demonstrates doing a push request to update a database. I had to create a backend in order to post this on Netlify. The App makes a get request to the backend then displays the movie data. Different routes are used so that the user can focus on one movie or edit a movie listing. If the user goes to a movie page, they can then add it to their saved list. The can click edit which goes to another page with a form for editing. Once submitted, a put request is sent and the backend is updated. This project demonstrates:
This was a team project. I worked on the front end while my partner created the back end. This app allows users to register a new account. They can choose a user name and password. A student can then log into the student page and view tickets submitted by others, tickets that they have submitted, and also create a new help ticket. The helper can also view tickets that have been submitted. They can also assign tickets to themselves. After they do this, they can view tickets that they have assigned to themselves. They can also make a ticket as resolved as well as return a ticket to the queue. (User name aaron and password is pass.) This project demonstrates:
For this project, I was provided a database. I created a server.js file and a router.js file to handle API requests. I used express as my Node web framework and wrote code for an API with seven different end points. This project demonstrates:
A local Ophthalmology company needed a redesign of their webpage. Some users had difficulty reading the page because the fonts were too small. Also, the webpage was not designed to by viewed on mobile devises. The orginal webpage can be found here. Unfortunately, the company has gone out of business but I have posted the redesign approved by the companies tech supervisor. This project demonstrates:
Our final team project, which lasted for several weeks. We had a team of six working on this task organizer that included a project manager and two IOS developers. The rest of us were web developers that were continuing a project that was started by another team. One of my partners worked on the front-end exclusively while the other worked on the back-end exclusively. Most of my work was also on the front-end but I also helped out on the back-end. As a team, we created a Notion document for our product board. Part of this work included creating release canvases. We created versions 1.1 - 1.3 and completed 1.1 and 1.2. I was assigned to work on:
On our Trello Board
(page 2), you can see that I also
did work on the introduction page and the footer.
To login, use the email address is bob1@gmail.com and the password is 1234test.
This project demonstrates:
This website itself is a personal project. This is a simple static website that acts like a landing page. This project demonstrates:
I created this React based front-end as part of a job application. I was asked to pull data from the NASA api and display it on a page. They also wanted to see a like button where you could toggle between like and unliking. The button did not have to save state but they did want me to style that page and add an extra feature. I chose to do the 'change date' feature so that the user can look at past NASA images. This project demonstrates:
Betting on sports is a hobby of mine which I do for fun. I wanted to see how successful my strategies would be if I bet on NBA games over an entire season. In order to do this, I would first need a database with all games stats and betting lines. I therefore created a Python Flask back-end with a relational database. I used the Python package Beautiful Soup to scrape the data I needed and store it into my database. Next I created a front-end with React. The front-end would collect the data, calculate handicaps, decide whether to bet or not based on those handicaps, and then return the results. This project demonstrates:
* Extra work that was not required.